模型关系(Relationships between Models)¶
有四种关系类型:1对1,1对多,多对1,多对多。关系可以是单向或者双向的,每个关系可以是简单的(一个1对1的模型)也可以是复杂的(一组多个模型)。
单向关系(Unidirectional relationships)¶
单向关系在两个模型中的一个模型中定义了彼此的关系。
双向关系(Bidirectional relations)¶
双向关系在两个模型中建立关系,每个模型定义了另一个模型的反向关系。
定义关系(Defining relationships)¶
在Phalcon中,关系必须在模型的代码:initialize())方法中定义。方法:code:`belongsTo(),:code:hasOne(),
:code:hasMany()`和:code:`hasManyToMany()`定义当前模型中一个或多个字段之间的关系,
另一个模型。这些方法中的每一个都需要3个参数:局部字段,引用模型,引用字段。
在Phalcon中,关系必须在模型的 :code:`initialize() 方法中定义。
可以使用方法 belongsTo()
、hasOne()
、hasMany()
以及 hasManyToMany()
定义当前模型和另外一个模型中一个或多个字段之间的关系。
这些方法中的每一个都需要3个参数:local fields(当前模型字段), referenced model(引用模型), referenced fields(引用字段)。
Method | Description |
---|---|
hasMany | Defines a 1-n relationship |
hasOne | Defines a 1-1 relationship |
belongsTo | Defines a n-1 relationship |
hasManyToMany | Defines a n-n relationship |
The following schema shows 3 tables whose relations will serve us as an example regarding relationships:
CREATE TABLE `robots` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(70) NOT NULL,
`type` varchar(32) NOT NULL,
`year` int(11) NOT NULL,
PRIMARY KEY (`id`)
);
CREATE TABLE `robots_parts` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`robots_id` int(10) NOT NULL,
`parts_id` int(10) NOT NULL,
`created_at` DATE NOT NULL,
PRIMARY KEY (`id`),
KEY `robots_id` (`robots_id`),
KEY `parts_id` (`parts_id`)
);
CREATE TABLE `parts` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(70) NOT NULL,
PRIMARY KEY (`id`)
);
- The model “Robots” has many “RobotsParts”.
- The model “Parts” has many “RobotsParts”.
- The model “RobotsParts” belongs to both “Robots” and “Parts” models as a many-to-one relation.
- The model “Robots” has a relation many-to-many to “Parts” through “RobotsParts”.
Check the EER diagram to understand better the relations:
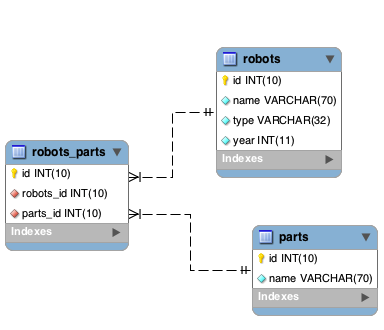
The models with their relations could be implemented as follows:
<?PHP
use Phalcon\Mvc\Model;
class Robots extends Model
{
public $id;
public $name;
public function initialize()
{
$this->hasMany("id", "RobotsParts", "robots_id");
}
}
<?php
use Phalcon\Mvc\Model;
class Parts extends Model
{
public $id;
public $name;
public function initialize()
{
$this->hasMany("id", "RobotsParts", "parts_id");
}
}
<?php
use Phalcon\Mvc\Model;
class RobotsParts extends Model
{
public $id;
public $robots_id;
public $parts_id;
public function initialize()
{
$this->belongsTo("robots_id", "Robots", "id");
$this->belongsTo("parts_id", "Parts", "id");
}
}
The first parameter indicates the field of the local model used in the relationship; the second indicates the name of the referenced model and the third the field name in the referenced model. You could also use arrays to define multiple fields in the relationship.
Many to many relationships require 3 models and define the attributes involved in the relationship:
<?php
use Phalcon\Mvc\Model;
class Robots extends Model
{
public $id;
public $name;
public function initialize()
{
$this->hasManyToMany(
"id",
"RobotsParts",
"robots_id", "parts_id",
"Parts",
"id"
);
}
}
使用关系(Taking advAntage of relationships)¶
When explicitly defining the relationships between models, it is easy to find related records for a particular record.
<?php
$robot = Robots::findFirst(2);
foreach ($robot->robotsParts as $robotPart) {
echo $robotPart->parts->name, "\n";
}
Phalcon uses the magic methods __set
/__get
/__call
to store or retrieve related data using relationships.
By Accessing an attribute with the same name as the relationship will retrieve all its related record(s).
<?php
$robot = Robots::findFirst();
$robotsParts = $robot->robotsParts; // All the related records in RobotsParts
Also, you can use a magic getter:
<?php
$robot = Robots::findFirst();
$robotsParts = $robot->getRobotsParts(); // All the related records in RobotsParts
$robotsParts = $robot->getRobotsParts(array('limit' => 5)); // Passing parameters
If the called method has a “get” prefix Phalcon\Mvc\Model will return a
findFirst()
/find()
result. The following example compares retrieving related results with using magic methods
and without:
<?php
$robot = Robots::findFirst(2);
// Robots model has a 1-n (hasMany)
// relationship to RobotsParts then
$robotsParts = $robot->robotsParts;
// Only parts that match conditions
$robotsParts = $robot->getRobotsParts("created_at = '2015-03-15'");
// Or using bound parameters
$robotsParts = $robot->getRobotsParts(
array(
"created_at = :date:",
"bind" => array(
"date" => "2015-03-15"
)
)
);
$robotPart = RobotsParts::findFirst(1);
// RobotsParts model has a n-1 (belongsTo)
// relationship to RobotsParts then
$robot = $robotPart->robots;
Getting related records manually:
<?php
$robot = Robots::findFirst(2);
// Robots model has a 1-n (hasMany)
// relationship to RobotsParts, then
$robotsParts = RobotsParts::find("robots_id = '" . $robot->id . "'");
// Only parts that match conditions
$robotsParts = RobotsParts::find(
"robots_id = '" . $robot->id . "' AND created_at = '2015-03-15'"
);
$robotPart = RobotsParts::findFirst(1);
// RobotsParts model has a n-1 (belongsTo)
// relationship to RobotsParts then
$robot = Robots::findFirst("id = '" . $robotPart->robots_id . "'");
The prefix “get” is used to find()
/findFirst()
related records. Depending on the type of relation it will use
‘find’ or ‘findFirst’:
Type | Description | Implicit Method |
---|---|---|
Belongs-To | Returns a model instance of the related record directly | findFirst |
Has-One | Returns a model instance of the related record directly | findFirst |
Has-Many | Returns a collection of model instances of the referenced model | find |
Has-Many-to-Many | Returns a collection of model instances of the referenced model, it implicitly does ‘inner joins’ with the involved models | (complex query) |
You can also use “count” prefix to return an integer denoting the count of the related records:
<?php
$robot = Robots::findFirst(2);
echo "The robot has ", $robot->countRobotsParts(), " parts\n";
定义关系(Aliasing Relationships)¶
To explain better how aliases work, let’s check the following example:
The “robots_similar” table has the function to define what robots are similar to others:
mysql> desc robots_similar;
+-------------------+------------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------------------+------------------+------+-----+---------+----------------+
| id | int(10) unsigned | NO | PRI | NULL | auto_increment |
| robots_id | int(10) unsigned | NO | MUL | NULL | |
| similar_robots_id | int(10) unsigned | NO | | NULL | |
+-------------------+------------------+------+-----+---------+----------------+
3 rows in set (0.00 sec)
Both “robots_id” and “similar_robots_id” have a relation to the model Robots:
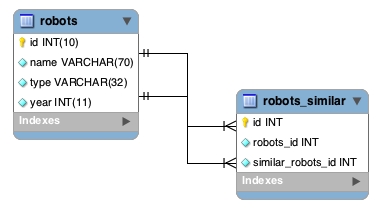
A model that maps this table and its relationships is the following:
<?php
class RobotsSimilar extends Phalcon\Mvc\Model
{
public function initialize()
{
$this->belongsTo('robots_id', 'Robots', 'id');
$this->belongsTo('similar_robots_id', 'Robots', 'id');
}
}
Since both relations point to the same model (Robots), obtain the records related to the relationship could not be clear:
<?php
$robotsSimilar = RobotsSimilar::findFirst();
// Returns the related record based on the column (robots_id)
// Also as is a belongsTo it's only returning one record
// but the name 'getRobots' seems to imply that return more than one
$robot = $robotsSimilar->getRobots();
// but, how to get the related record based on the column (similar_robots_id)
// if both relationships have the same name?
The aliases allow us to rename both relationships to solve these problems:
<?php
use Phalcon\Mvc\Model;
class RobotsSimilar extends Model
{
public function initialize()
{
$this->belongsTo(
'robots_id',
'Robots',
'id',
array(
'alias' => 'Robot'
)
);
$this->belongsTo(
'similar_robots_id',
'Robots',
'id',
array(
'alias' => 'SimilarRobot'
)
);
}
}
With the aliasing we can get the related records easily:
<?php
$robotsSimilar = RobotsSimilar::findFirst();
// Returns the related record based on the column (robots_id)
$robot = $robotsSimilar->getRobot();
$robot = $robotsSimilar->robot;
// Returns the related record based on the column (similar_robots_id)
$similarRobot = $robotsSimilar->getSimilarRobot();
$similarRobot = $robotsSimilar->similarRobot;
魔术方法 Getters 对比显示方法(Magic Getters vs. Explicit methods)¶
Most IDEs and editors with auto-completion capabilities can not infer the correct types when using magic getters, instead of use the magic getters you can optionally define those methods explicitly with the corresponding docblocks helping the IDE to produce a better auto-completion:
<?php
use Phalcon\Mvc\Model;
class Robots extends Model
{
public $id;
public $name;
public function initialize()
{
$this->hasMany("id", "RobotsParts", "robots_id");
}
/**
* Return the related "robots parts"
*
* @return \RobotsParts[]
*/
public function getRobotsParts($parameters = null)
{
return $this->getRelated('RobotsParts', $parameters);
}
}
虚拟外键(Virtual Foreign Keys)¶
By default, relationships do not act like database foreign keys, that is, if you try to insert/update a value without having a valid value in the referenced model, Phalcon will not produce a validation message. You can modify this behavior by adding a fourth parameter when defining a relationship.
The RobotsPart model can be changed to demonstrate this feature:
<?php
use Phalcon\Mvc\Model;
class RobotsParts extends Model
{
public $id;
public $robots_id;
public $parts_id;
public function initialize()
{
$this->belongsTo(
"robots_id",
"Robots",
"id",
array(
"foreignKey" => true
)
);
$this->belongsTo(
"parts_id",
"Parts",
"id",
array(
"foreignKey" => array(
"message" => "The part_id does not exist on the Parts model"
)
)
);
}
}
If you alter a belongsTo()
relationship to act as foreign key, it will validate that the values inserted/updated on those fields have a
valid value on the referenced model. Similarly, if a hasMany()
/hasOne()
is altered it will validate that the records cannot be deleted
if that record is used on a referenced model.
<?php
use Phalcon\Mvc\Model;
class Parts extends Model
{
public function initialize()
{
$this->hasMany(
"id",
"RobotsParts",
"parts_id",
array(
"foreignKey" => array(
"message" => "The part cannot be deleted because other robots are using it"
)
)
);
}
}
A virtual foreign key can be set up to allow null values as follows:
<?php
use Phalcon\Mvc\Model;
class RobotsParts extends Model
{
public $id;
public $robots_id;
public $parts_id;
public function initialize()
{
$this->belongsTo(
"parts_id",
"Parts",
"id",
array(
"foreignKey" => array(
"allowNulls" => true,
"message" => "The part_id does not exist on the Parts model"
)
)
);
}
}
级联与限制动作(Cascade/Restrict actions)¶
Relationships that act as virtual foreign keys by default restrict the creation/update/deletion of records to maintain the integrity of data:
<?php
namespace Store\Models;
use Phalcon\Mvc\Model;
use Phalcon\Mvc\Model\Relation;
class Robots extends Model
{
public $id;
public $name;
public function initialize()
{
$this->hasMany(
'id',
'Store\\Models\\Parts',
'robots_id',
array(
'foreignKey' => array(
'action' => Relation::ACTION_CASCADE
)
)
);
}
}
The above code set up to delete all the referenced records (parts) if the master record (robot) is deleted.
在结果集中操作(Operations over Resultsets)¶
If a resultset is composed of complete objects, the resultset is in the ability to perform operations on the records obtained in a simple manner: